Simplifying Component Logic in React with Higher-Order Components

Posted By
Rasika Shinde
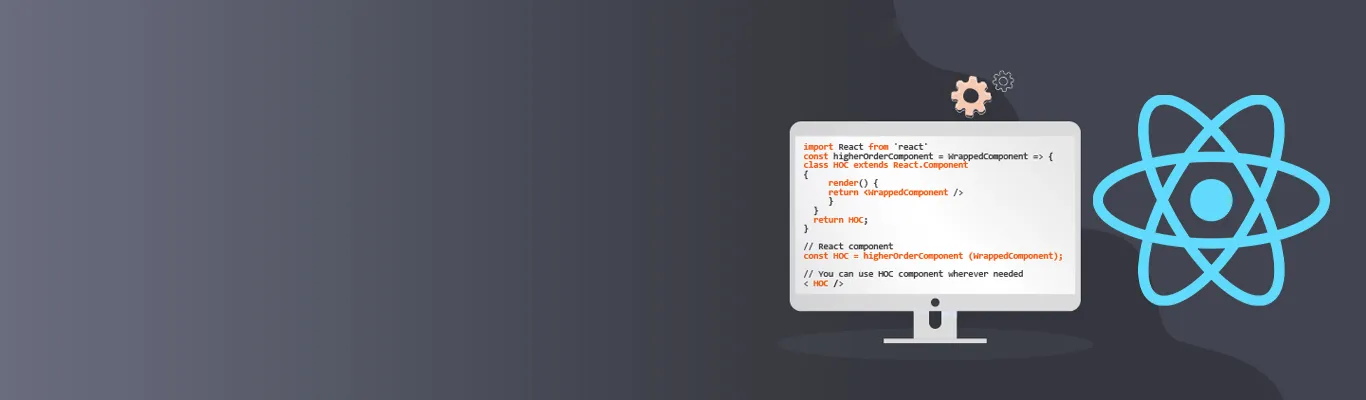
In one of my earlier blogs Enriching UI with Styled Components in React, I wrote about stylizing components in React. This time I am going to write about Higher-Order Components in React. Components act as one of the core building blocks in React. If you are a React user, you might have felt the need to have copies of the same logic in multiple components. However, React has an advanced technique to share logic across multiple components without the need to rewrite it. A Higher-Order Component (HOC) is a cutting-edge technique in React for reusing component logic. HOCs are not part of the React API, but it is a pattern that emerges from React’s compositional nature. HOC accepts the component and returns a new component.
HOCs are great while building React applications. They are similar to JavaScript functions and are used to inject additional functionality into existing components. They are also helpful in implementing the separation of concerns in components in a practical way.
Let me explain this with an example -
/// Definition of Higher Order Component import React from 'react' const higherOrderComponent = WrappedComponent => { class HOC extends React.Component { render() { return <WrappedComponent /> } } return HOC; }
/// Usage of Higher Order Component // React component const HOC = higherOrderComponent (WrappedComponent); // You can use HOC component wherever needed < HOC />
In the above example, Higher-Order Component is a function that uses a component named WrappedComponent as an argument. The new component created is called HOC, which returns the from its render function.
HOCs simply use an original component and return the enhanced component. Higher-Order Components are JavaScript functions that merge additional functionality to the existing component. These functions simply receive data and return the values according to that data. If you want to update the returning component, you just need to change the function's data.
/// Definition of Higher Order Component with modification of prop age import React from 'react'; const Enhancer = (WrappedComponent) => { class HOC extends React.Component { render() { return (<WrappedComponent {...this.props} age={42}/> ); } } return HOC; }; export default Enhancer;
/// Using Higher Order Component with Enhancer import React from 'react';, import Enhancer from 'components/withUserAge’; class DisplayTheAge { render() { return <div>User’s age is {this.data}</div>; } } export default Enhancer(DisplayTheAge);
Here WrappedComponent is just an enhanced version of <DisplayTheAge/>, facilitating the access age as a prop. You can use the Higher-Order Component at multiple places with different sets of data. Here Enhancer is an enhancer class for WrappedComponent. This allows users to fulfill the actual use of Higher-Order Components.
Following are the best practices, methods, and techniques in React that would help you maintain consistency during application development.
- Render Hijacking
Render hijacking is the ability to control what a component output will be from another component. You decorate their components by wrapping them into a Higher-Order Component. By wrapping, you can inject additional props or make other changes, which can cause changing logic of rendering. HOCs take control of the render output of the WrappedComponent and can manipulate it.
In Render Hijacking, you can read, add, edit or remove the props coming from the output component in HOC. Also, you can choose to display the inner element conditionally.
- State & Props Manipulation
The HOCs can read, edit and delete the state of the WrappedComponent instance, and you can also add more states if you want to. But ideally, HOCs should be limited to read or add state for avoiding breaking the implementation of the things.
- Inheritance Inversion (iiHOC)
The returned Enhancer class extends the WrappedComponent. It is called Inheritance Inversion because instead of the WrappedComponent extending some Enhancer class, it is extended by the Enhancer. The relationship between them looks reversed
function iiHOC(WrappedComponent) {, return class Enhancer extends WrappedComponent { render() { return super.render() } } }
React is a library that builds composable user interfaces. It also creates reusable UI components that present data changing over time. Higher-Order Components are primarily preferred to reuse logic in React apps. However, you will need to render some UI, which makes it inconvenient to use while sharing some non-visual logic. In such a case, React hooks come to the rescue and act as a perfect mechanism for code reuse.
Get in touch with our team for faster application development needs using reusable code with better performance and a simpler programming model.
Related Blogs
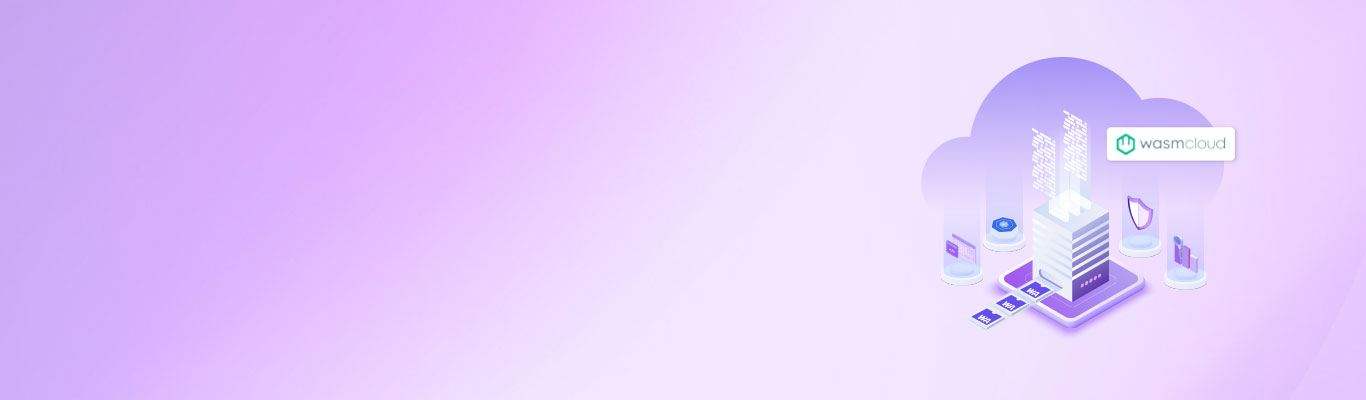
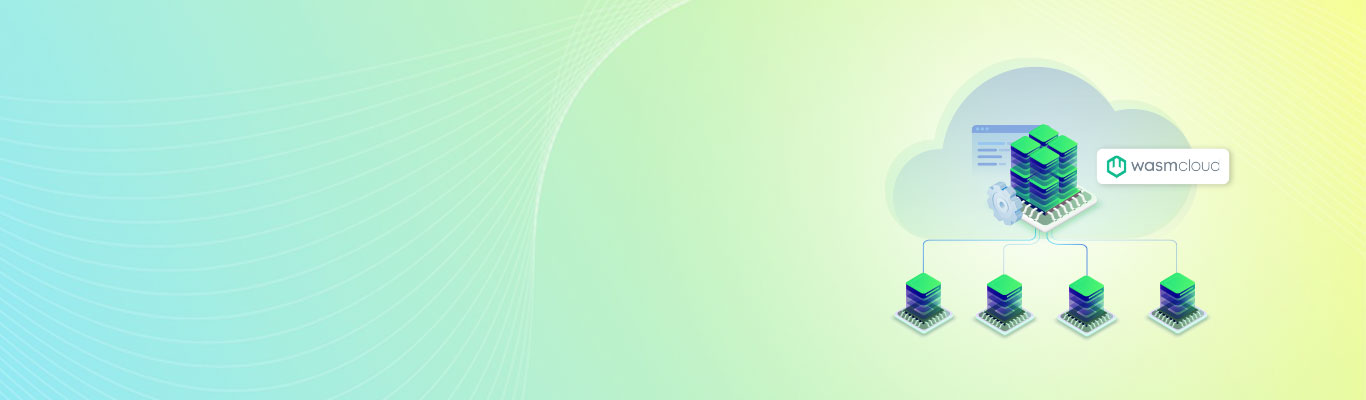
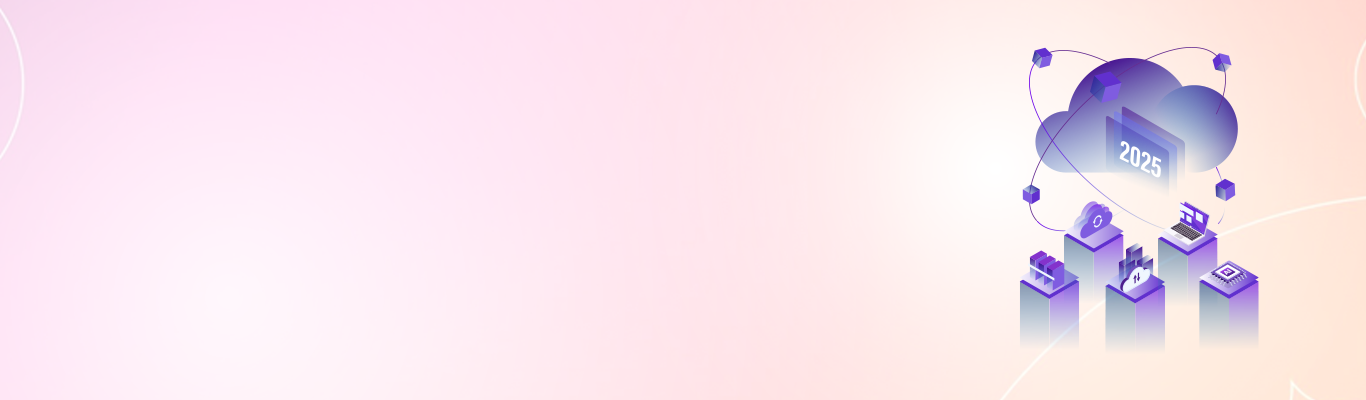
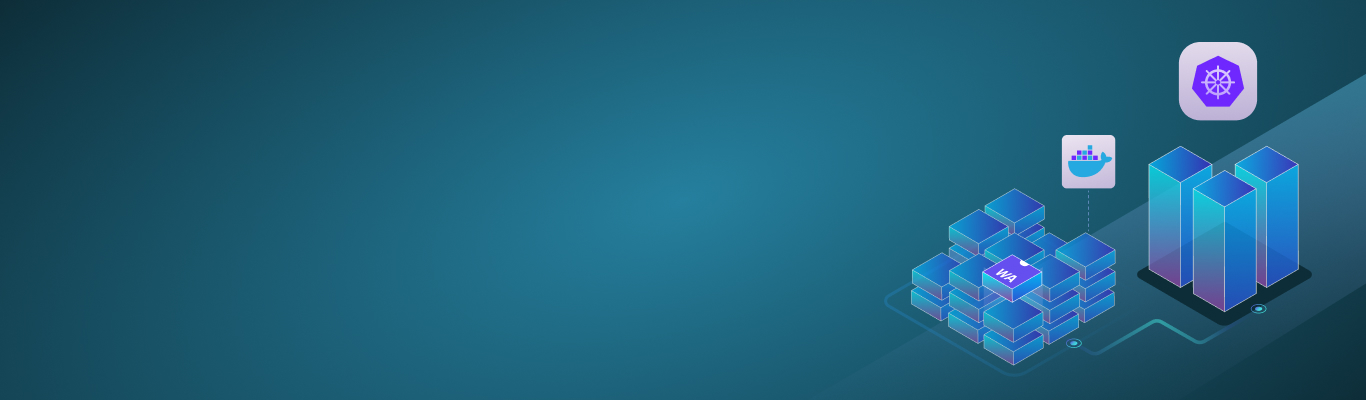