React JS Component Lifecycle Methods

Posted By
Chandan Jawale
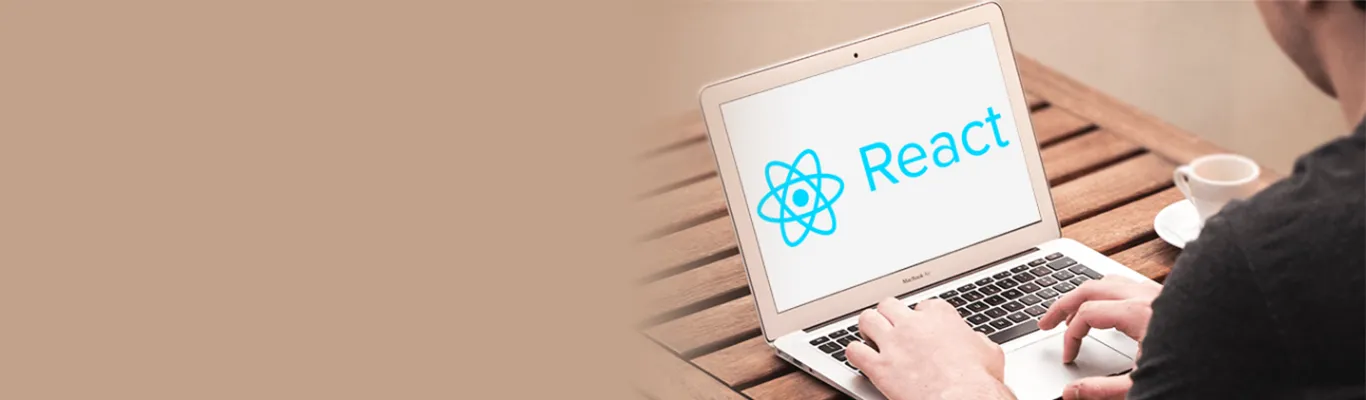
Introduction to React JS in short:
React JS is a JavaScript library for creating User Interfaces authored by Facebook and Instagram engineers. React JS is all about components. Each component lets you split the User Interface (UI) into independent, reusable pieces and allows you to think about each piece in isolation.
One of the most important features of React JS, which makes it stand out, is that it allows you to simply express how your app should look based on your data at any point in time. When data changes, React JS automatically handles all the changes in the UI without you having to deal with Document Object Model (DOM). React JS uses a fast, in-memory, and lightweight representation of DOM called virtual DOM. React JS basically uses a very optimized diff algorithm that compares the virtual DOM of the current and previous state.
Component Lifecycle:
Before we dive deep into the React Component lifecycle, I would like you to think about your lifecycle and then compare both - your and React JS lifecycle. Every lifecycle signifies one important thing “CHANGE.” Everything is constantly changing over time. From the moment you’re born, you start changing till your last breath. You can divide your life into parts that you can call the stages or the phases of life. The same is the case for React Component, and once you understand the similarity, it will be very easy for you to understand the React JS Component Lifecycle and its various methods during the different stages/phases.
React Component Lifecycle Methods and Benefits:
The component lifecycle methods are various methods that are invoked at different phases of the lifecycle of a component. Consider a scenario where you are creating an application in which you can allow multiple videos to run on the same page. In this case, the application will use the network to buffer videos and it will keep on consuming battery. But if you add the React Component for each video, you will be able to play/pause the video, which will make sure only those videos are played that you want to watch. As a result, you will save battery as well as network usage. You can decide whether to play the video or not as you hover over, based on your choice. And you can do this with the help of React Component lifecycle methods.
So, with the use of lifecycle methods provided by React JS, developers can produce a quality application and can really plan what and how at various points of birth, growth, or death of user interfaces.
You can take your React JS User Interfaces to the next level in a short time if you combine your development skills with the component lifecycle.
Phases of React Component Lifecycle
Each React Component goes through different stages of lifecycle that are called Phases in React terminology. All React Component Lifecycle methods can be split into four phases of React Component:
1. Initialization
2. Mounting
3. Updating
4. Unmounting
The process in which all these phases are involved is called the component’s lifecycle. Each and every React Component goes through the component’s lifecycle. React JS provides several methods that notify us when a certain stage of this process occurs. These methods are called the component’s lifecycle methods, and they are invoked in a predictable order.
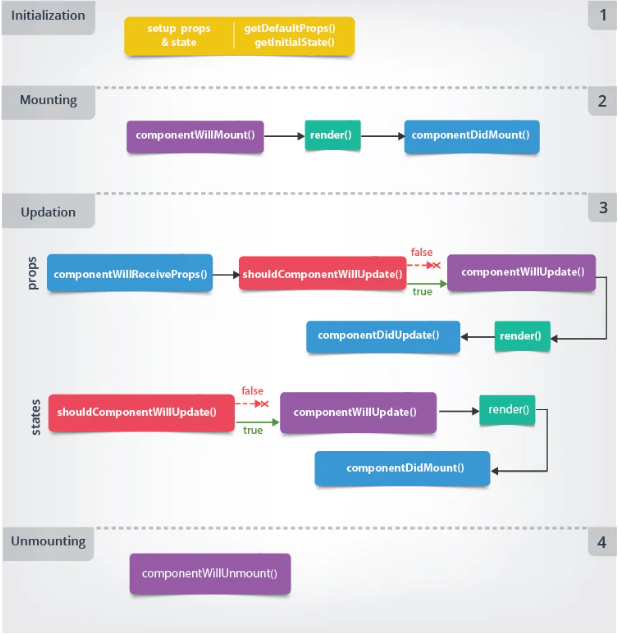
Let’s take a closer look at each phase of React Component:
1. Initialization (refer to section 1 in the image)
The first phase is Initialization, where you can define defaults and initial values for this.props and this.state by implementing getDefaultProps() and getInitialState() methods, respectively. Both methods are called before the Mounting phase starts.
The getDefaultProps can be used to define any default props that can be accessed using this.props.
getDefaultProps (){ console.log('1. getDefaultProps'); return { /* something here */}; }
The getInitialState method enables us to set the initial value for the state which is accessible inside the component using this.state.
getInitialState (){ console.log('2. getInitialState'); return { /* something here */}; };
The render function gets called after the getDefaultProps and getInitialState functions
render () { console.log('3. render'); return ( <div> ... </div> ) };
If you add and run these three functions in one component, then you will be able to see the logs statement in a console as follows:
i) getDefaultProps
ii) getInitialState
iii) render
2. Mounting (refer to section 2 in the image)
After preparing the basic needs, states, and props, your component gets ready to mount in browser DOM. In other words, Mounting is the process that occurs when a component is inserted into the DOM.
This phase has two methods that you can associate with:
i) componentWillMount()
This Method gets called before the render method is executed. It is important to note that setting the state in this phase will not trigger a re-rendering. And at the end of this method, the component will mount. All the things that you want to do before a component mounts have to be defined here.
This method can be used for initializing state and props.
It’s very important to note that calling this.setState() within this method will not trigger a re-render.
This method is executed once in a lifecycle of a component and before the first render.
componentWillMount() { console.log("componentWillMount"); }
ii) componentDidMount (refer to section 2 in the image)
This method gets called after the render method has been executed. The DOM can be accessed, which enables you to define DOM manipulations or data fetching operations. Any DOM interactions should always happen in this phase and not inside the render method.
This method is executed once in a lifecycle of a component and after the first render.
componentDidMount() { console.log("componentDidMount"); }
If you add and run these three functions in one component, then you will be able to see the logs statement in a console as follows:
i) getDefaultProps
ii) getInitialState
iii) componentWillMount
iv) render
v) componentDidMount
3. Updating (refer to section 3 in the image)
In this phase, the methods are called when a component’s state or properties get updated, and the component is re-rendered.
During this phase, a React Component is already inserted into the DOM. Thus, these methods are not called for the first render.
These methods are called in the following way as per the change in state or props:
A) When receiving a new prop
i) componentWillReceiveProps
ii) shouldComponentUpdate (If this method returns true, then the next method will get called; otherwise breaks here)
iii) componentWillUpdate
iv) render
v) componentDidMount
Any changes on the props object will also trigger the lifecycle and are almost identical to the state change with one additional method being called.
i) componentWillReceiveProps
This method is called whenever a component receives new props and when it is not in initial rendering. This method enables us to update the state depending on the existing and upcoming props without triggering another rendering. One interesting thing to remember here is that there is no equivalent method for the state as state changes should never trigger any props changes.
Note: This method is called several times. So, it is better to compare new props with old ones.
componentWillReceiveProps: function(nextProps) { this.setState({ // set something }); }
ii) shouldComponentUpdate
This method always gets called before the render method. In this method, we can decide whether re-rendering the component is necessary or not. To do this, a boolean value must be returned.
This method is never called on initial rendering.
shouldComponentUpdate(nextProps, nextState){ // return a boolean value return true; }
iii) componentWillUpdate
This method will be called after shouldComponentUpdate method returns true. This method does the preparation for the upcoming render similar to the componentWillMount. There can be some use cases where you may need some calculation or preparation before rendering some item; this is the place to do so.
iv) render
In this method, the updated component will be rendered on the screen with updated data or changes.
v) componentDidUpdate
This method gets called after the render method. This is similar to the componentDidMount and can be used to perform DOM operations after the data has been updated.
componentDidUpdate(prevProps, prevState){ console.log('componentDidUpdate’); }
B. When state changes via this.setState()
i) shouldComponentUpdate (If this method returns true, then the next method will get called; otherwise breaks here)
ii) compoentWillUpdate
iii) render
iv) componentDidUpdate
Any changes in the state will also trigger the lifecycle and are almost the same as changes in props, with one less method being called.
The rest of the lifecycle reveals nothing new here and is identical to the state change triggered cycle.
4. Unmounting (refer to section 4 in the image)
This is the last phase of a component, where the component dies or gets destroyed.
Only one componentWillUnmount method gets called in this phase.
This method gets called before the component is removed from the DOM and can be used to perform cleanup operations.
Right things done with right skills and intentions at right time generate the right results compared to the ones where any one of the mentioned components is missing, especially the time. The same thing applies here, that if you follow the React Component lifecycle and use the proper methods, you will have a component with great performance and fewer bugs.
React provides you an option to declare methods that will be called automatically, while creating components, in certain occasions throughout the component’s lifecycle. The significance of each component’s lifecycle method and the order in which they are called gives you special privileges to perform certain actions while the component is created or destroyed and facilitates third-party library integrations and performance optimization.
This was just to give you a quick idea of React JS and React Component lifecycle phases. We will be back with the actual react app creation with react-bootstrap details in part 2 of this blog. Stay tuned!